Mary Had a Little Lambda
Originally I set out to write a sequel to Functional Programming Fundamentals that covered all of the scary jargon. This quickly evolved into What the Functor? In that article I covered monads, monoids, and functors. Now we have one last bit of scary functional jargon, lambdas.
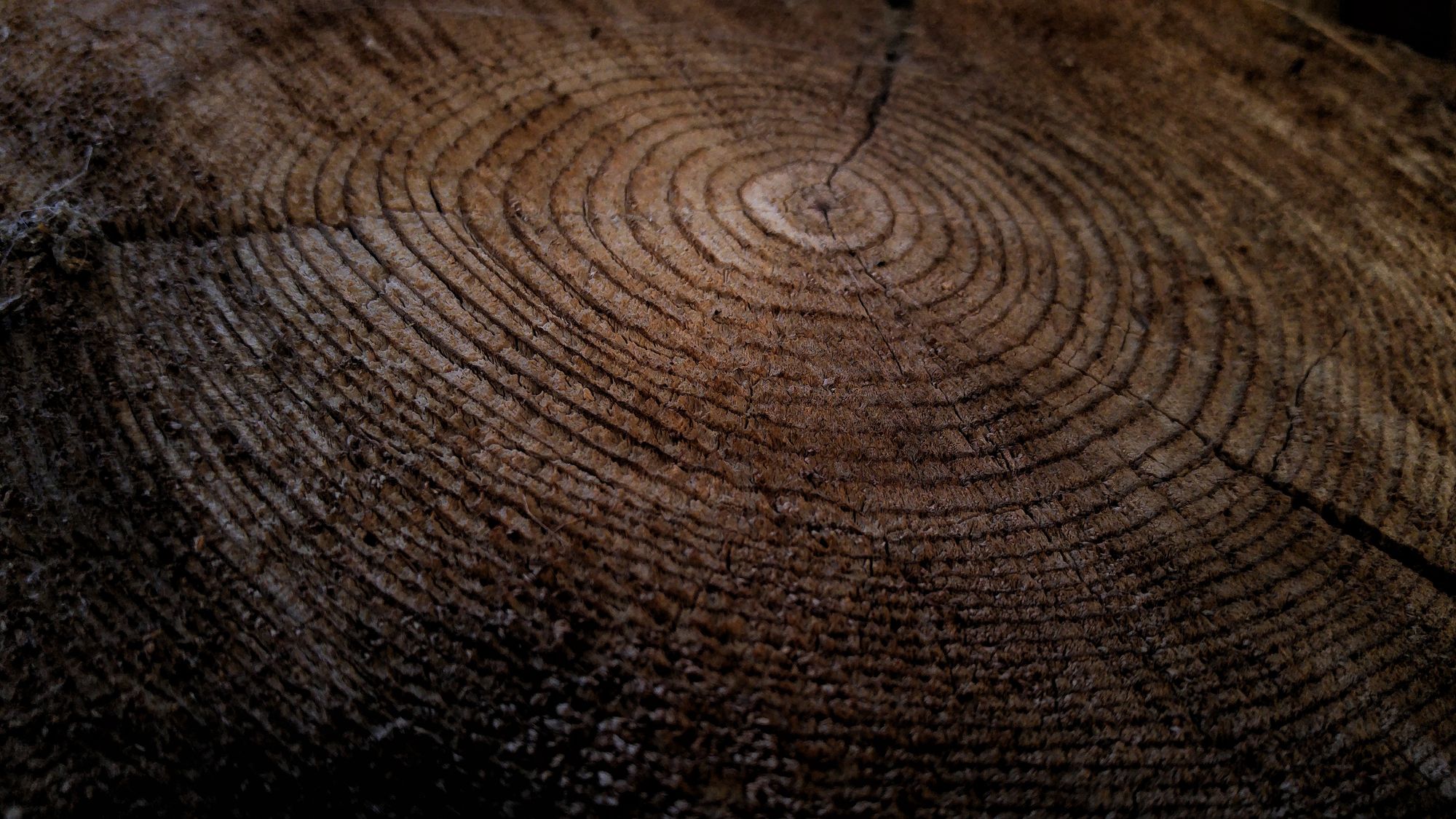
Originally I set out to write a sequel to Functional Programming Fundamentals that covered all of the scary jargon. This quickly evolved into What the Functor?
In that article I covered monads, monoids, and functors. Now we have one last bit of scary functional jargon, lambdas.
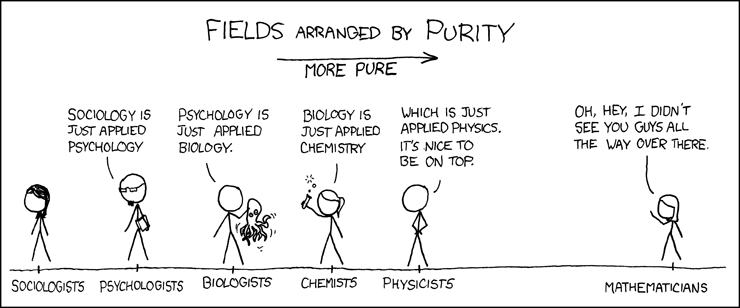
Lambda
A lambda is an anonymous function that can be treated like a value. [2]
We actually saw some of these in the previous post. These were learnExpelliarmous
and joinGryffindor
.
In this case we create an anonymous function and assign it to the variable joinGryffindor
. We then pass this as an argument to map
. We could also just pass this anonymous function directly like so:
This is a common pattern, passing a lambda, or an anonymous function to a higher order function.
Lambda Calculus
Now the scarier term Lambda Calculus. Let's break this down. Lambdas are anonymous functions, we just learned that.
Why don't we define calculus now? If we pull up the dictionary definition we get the following
A method of computation or calculation in a special notation (as of logic or symbolic logic. [3]
Lambda Calculus is just a formal way of defining functions using special notation. Less scary now, right?
Let's dive just a little more into it. Lambda Calculus, or the formal notation for defining functions, is composed of three elements variables, functions, and applications. [4]
I'm going to go ahead and heavily paraphrase this great article from learn x in y minutes.
Variables
We know these, variables are used in programming all the time, and we can name them anything. Lets go with one named x
. [4]
Function
We know these too! We use functions in our code, and that's why you're reading an article on functional programming. In lambda calculus they use a special syntax: λ<parameters>.<body>
. As an example λx.x
is the identity function. [4]
Application
This is a new word, but we see this all the time too. This is the act of calling a function. So if a function is the function declaration, this is the actual function call.
The syntax for this even involves parentheses! (λx.x)a
calls the identity function with the argument a
.
Here's a useful table taken from the article mentioned above. [4]
Name | Syntax | Example | Explanation |
---|---|---|---|
Variable | <name> |
x |
a variable named “x” |
Function | λ<parameters>.<body> |
λx.x |
a function with parameter “x” and body “x” |
Application | <function><variable or function> |
(λx.x)a |
calling the function “λx.x” with argument “a” |
This took me a while to grok. If you're looking for more resources I recommend Computerphiles excellent video on Lambda Calculus and the sequel on Y Combinators.
Predicate
Let's finish up with a comparatively straightforward term. What is a predicate? A predicate is just a function that returns true or false [5]. It's most commonly used as criteria for a filter.
Here's a predicate:
Wrapping Up
I'd like to reiterate, none of this is important to your day to day code. But if you were curious what all those scary FP terms mean hopefully this satisfied that curiosity.
If you'd like something that will make your code better, check out Functional Programming Fundamentals. If you want even scarier terms check out What the Functor?
Also if you feel the need to buy me a drink you can do so here or follow me on twitter @MatthewGerstman.
Ads
These ads help me pay to keep this site up. Feel free to buy, watch, listen or ignore these like any other ad.
References
- https://www.youtube.com/watch?v=eis11j_iGMs
- https://github.com/hemanth/functional-programming-jargon
- https://www.merriam-webster.com/dictionary/calculus
- https://learnxinyminutes.com/docs/lambda-calculus/
- https://stackoverflow.com/questions/3230944/what-does-predicate-mean-in-the-context-of-computer-science