Map, Filter, Reduce
In this article we'll go back to basics and cover three of the most important functions in functional programming, map, filter, and reduce.
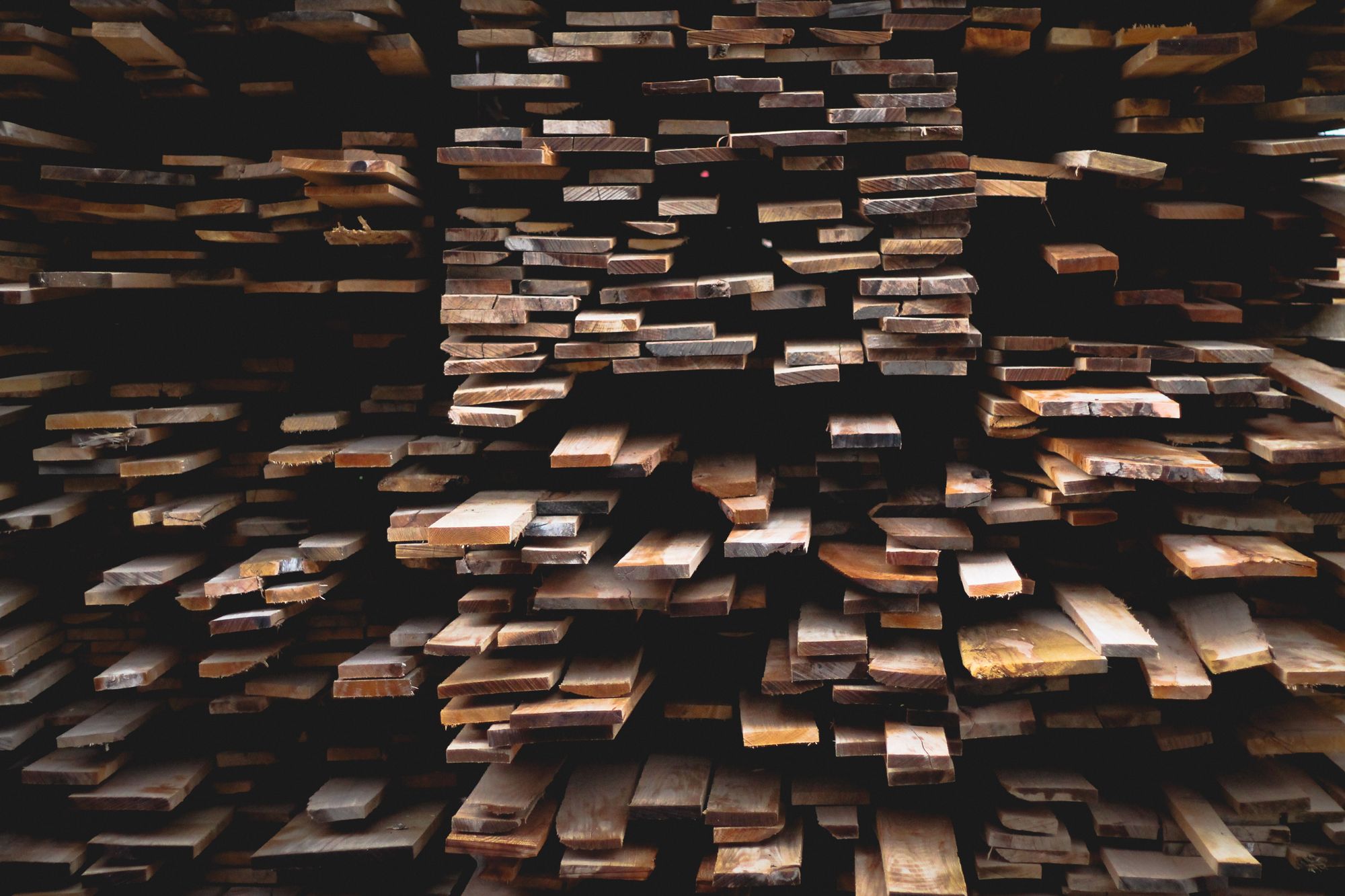
In this article we'll go back to basics and cover three of the most important functions in functional programming, map, filter, and reduce.
All three of these are useful functions for iterating over a list (or array) and doing some kind of transformation or computation. This will then produce a new list or the result of the computation done across the previous list.
Types
Before we dive into map, filter, and reduce, let's set up the list.
As per usual, we're going to be operating on wizards. Each wizard object has a name, a house, and the number of points they've earned for their house. Let's declare a bunch of wizards and put them in a list.
Map
Now that we've got the boilerplate out of the way, let's dive in. The first function is the simplest one, map. Map iterates (or loops) over a list, applies a function to each item in that list and then returns a new list of the transformed items. Let's look at an example.
This function iterates over the list of wizards, gets their name, and puts it into a new array. The result of this looks something like this.
Now in this example we were using a lambda (aka an anonymous function), but we can also use a named function.
In this example we have a function called wizardToString
and we pass that directly to map. It will then return a new list that looks like this.
Filter
Next up is filter. Filter behaves like map in that it iterates over the list, but it instead of transforming each item, it transforms the whole list. Filter takes a function that returns true or false or predicate. It then returns a new list with items where the predicate returns true
. Let's look at an example.
In this example we filter over the list and only include wizards who are in the house Slytherin. The result would be this.
As an aside, Taylor and Lin are two of the most acclaimed Slytherins of our day.
Just like with map, we don't need to use a lambda, we can also use a predefined function.
In this example we make two lists, the list of Slytherins who have earned points (winners), and the list of Slytherins who have lost points (losers). We can see those results below.
Reduce
Now we get to the most interesting function, reduce. Reduce iterates over a list and produces a single value. Let's look at an example.
Suppose we want to get the total number of points across all wizards. We can use reduce to do that.
What's going on here? Well reduce is a function that takes two arguments, a function and an initial value for the accumulator. The accumulator is the name of the thing reduce returns. In this case we start our point count at 0.
Now the function takes the current state of the accumulator and the item in the list it's supposed to process. For the first wizard, it will pass 0 for the accumulator. This function then returns accumulator + points
. This will eventually sum up all the points. If you're curious, the result is 5487.
Now the accumulator can be anything, we can even use reduce to produce an object. Let's look at an example where we add up the points for each house.
In this case, we initialize our accumulator or acc
with {}
. Then for each wizard we call a function that adds the number of points that wizard has earned for their house. If you're curious that result looks like this.
Let's look at one more example, let's say we want the best wizard for each house. We can tweak our previous function to use the best wizard for each house.
If you're curious, the result of that looks like this.
Just a little more fun now, we can use Object.values
to transform this map.
Now we have nice strings for the best person in each house.
Wrapping Up
Hopefully you're more comfortable with map, filter, and reduce now. If you'd like a deeper dive into practical FP check out my article, Functional Programming Fundamentals.
If you're looking for more abstract stuff check out What the Functor? and Mary Had a Little Lambda.
Also if you feel the need to buy me a drink you can do so here or follow me on twitter @MatthewGerstman.